翻訳して勉強するGtkチュートリアル第11章は Spinner です。
Gtk.Spinner はアイコンサイズの回転アニメーションを表示します。これは、実際の進捗状況ではなく不定のアクティビティを表示するために、 GtkProgressBar の代わりとしてよく使われます。
アニメーションを開始するには Gtk.Spinner.start() を、停止するには Gtk.Spinner.stop() を使います。
11.1. 例
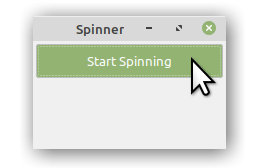
# tut11-01.py
# スピナー
import gi
gi.require_version("Gtk", "3.0")
from gi.repository import Gtk
class SpinnerAnimation(Gtk.Window):
def __init__(self):
Gtk.Window.__init__(self, title="Spinner")
self.set_border_width(3)
self.connect("destroy", Gtk.main_quit)
self.button = Gtk.ToggleButton("Start Spinning")
self.button.connect("toggled", self.on_button_toggled)
self.button.set_active(False)
self.spinner = Gtk.Spinner()
self.table = Gtk.Table(3, 2, True)
self.table.attach(self.button, 0, 2, 0, 1)
self.table.attach(self.spinner, 0, 2, 2, 3)
self.add(self.table)
self.show_all()
def on_button_toggled(self, button):
if button.get_active():
self.spinner.start()
self.button.set_label("Stop Spinning")
else:
self.spinner.stop()
self.button.set_label("Start Spinning")
myspinner = SpinnerAnimation()
Gtk.main()
11.2. 拡張例
回転アニメーションの開始と停止にタイムアウト関数を使用した拡張例です。on_timeout() 関数は、False を返すまで一定の間隔で呼び出され、その時点でタイムアウトは自動的に破棄され、関数が再び呼び出されることはありません。
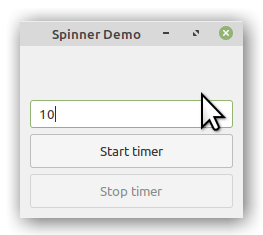
# tut11-02.py
# スピナー②
import gi
gi.require_version("Gtk", "3.0")
from gi.repository import Gtk, GLib
class SpinnerWindow(Gtk.Window):
def __init__(self, *args, **kwargs):
Gtk.Window.__init__(self, title="Spinner Demo")
self.set_border_width(10)
mainBox = Gtk.Box(orientation=Gtk.Orientation.VERTICAL, spacing=6)
self.add(mainBox)
self.spinner = Gtk.Spinner()
mainBox.pack_start(self.spinner, True, True, 0)
self.label = Gtk.Label()
mainBox.pack_start(self.label, True, True, 0)
self.entry = Gtk.Entry()
self.entry.set_text("10")
mainBox.pack_start(self.entry, True, True, 0)
self.buttonStart = Gtk.Button("Start timer")
self.buttonStart.connect("clicked", self.on_buttonStart_clicked)
mainBox.pack_start(self.buttonStart, True, True, 0)
self.buttonStop = Gtk.Button("Stop timer")
self.buttonStop.set_sensitive(False)
self.buttonStop.connect("clicked", self.on_buttonStop_clicked)
mainBox.pack_start(self.buttonStop, True, True, 0)
self.timeout_id = None
self.connect("destroy", self.on_SpinnerWindow_destroy)
def on_buttonStart_clicked(self, widget, *args):
""" Handles "clicked" event of buttonStart. """
self.start_timer()
def on_buttonStop_clicked(self, widget, *args):
""" Handles "clicked" event of buttonStop. """
self.stop_timer("Stopped from button")
def on_SpinnerWindow_destroy(self, widget, *args):
""" Handles destroy event of main window. """
# ensure the timeout function is stopped
if self.timeout_id:
GLib.source_remove(self.timeout_id)
self.timeout_id = None
Gtk.main_quit()
def on_timeout(self, *args, **kwargs):
""" A timeout function.
Return True to stop it.
This is not a precise timer since next timeout
is recalculated based on the current time.
"""
self.counter -= 1
if self.counter <= 0:
self.stop_timer("Reached time out")
return False
self.label.set_label("Remaining: " + str(int(self.counter / 4)))
return True
def start_timer(self):
""" Start the timer. """
self.buttonStart.set_sensitive(False)
self.buttonStop.set_sensitive(True)
# time out will check every 250 miliseconds (1/4 of a second)
self.counter = 4 * int(self.entry.get_text())
self.label.set_label("Remaining: " + str(int(self.counter / 4)))
self.spinner.start()
self.timeout_id = GLib.timeout_add(250, self.on_timeout, None)
def stop_timer(self, alabeltext):
""" Stop the timer. """
if self.timeout_id:
GLib.source_remove(self.timeout_id)
self.timeout_id = None
self.spinner.stop()
self.buttonStart.set_sensitive(True)
self.buttonStop.set_sensitive(False)
self.label.set_label(alabeltext)
win = SpinnerWindow()
win.show_all()
Gtk.main()